Open Kinect Prize
As stated in my 3D Vision for Embedded Systems post, Microsoft’s Kinect could be an extremely useful tool to give robots 3D vision. Now the fine people from Adafruit offer a prize for it. If you can provide open source software that gives access to the Kinect video signal (RGB) and distance values they will pay you 1000$. A tempting offer. I’m looking forward to seeing the results.
Update: The guys from ifixit.com got their hands on a Kinect and took it apart. The article shows detailed images of everything and even identifies all integrated circuit chips found inside the device.
Second update: Adafruit increased their offer to now $3000 and it looks like the problem isalready solved, at least partially. Check out this youtube video of the Kinect displaying RGB-D information on Windows 7. The depth information looks somewhat weird though. New updates can be found here. This video shows what the infrared projection of Kinect looks like. Pretty cool!
Third update: It is done! There now exists an open source driver for Microsoft’s Kinect. Adafruit announced the winner of the $3000. And more importantly the code can be found here.
Diffie-Hellman key exchange in Javascript
The Diffie-Hellman key exchange protocol (RFC2641) is an efficient way for two parties (that do not know each other beforehand) to establish a shared secret. This secret can then be used for encrypted communication between the two parties. Because of the simplicity of the algorithm it is a feasible to implement it in Javascript. It could serve as a way to communicate between to participants of a social network in a host-proof way (meaning the host can not access the messages that are exchanged between Alice and Bob).
The following straight forward implementation is based on the ingenious BigInt Javascript library created by Leemon Baird:
function d_h() { // Alice tries to send to Bob, they agree on a 256 bit prime var p = str2bigInt( "10883804965994030335610375728683210724614077" + "5791152305372594007835539736018383", 10, 80); var g = str2bigInt("2", 10, 80); // and on a generator // Alice chooses her secret randomly var a = str2bigInt( "3103830701216420526524336327874353922197141754" + "5159778383387464250", 10, 80); // Bob chooses his secret randomly var b = str2bigInt( "26264611999731746945409581849451656136966431" + "516270225144913718599547", 10, 80); // calculate the public key var A = powMod(g,a,p); var B = powMod(g,b,p); // A and B are exchanged publicly // Alice and Bob can compute the same key from A and B var a_sec = powMod(B, a, p); var b_sec = powMod(A, b, p); alert(bigInt2str(a_sec, 10) + '\n ' + bigInt2str(b_sec, 10)); }
In case you wonder how to choose p and g – so did I. Turns out a generator for those numbers is part of the Crypto++ library. The following application uses this library to calculate p and g with different length.
#include#include #include #include "dh.h" #include "osrng.h" using namespace std; using namespace CryptoPP; using namespace TCLAP; int main(int argc, char** argv) { // read command line CmdLine cmd("Diffie Hellman p g generator", ' ', "0.1"); UnlabeledMultiArg m("bv", "bits and values", true, ""); cmd.add( m ); cmd.parse(argc, argv); vector mv = m.getValue(); mv.push_back(1); unsigned int bv = mv[0]; unsigned int nv = mv[1]; AutoSeededRandomPool arngA; // generate p and g RandomNumberGenerator& rngA = *dynamic_cast (&arngA); for(unsigned short i=0; i The application uses the TCLAP library to parse the command line. The first parameter should be the width of the prime number in bits, the second optional parameter specifies how many primes and generators should be calculated.
Here's a list of p and g I generated with different widths:
128 bits: p=197221152031991558322935568090317202983 g=2 256 bits: p=11245712998331706449413325803449175679094351102802336690101496856041 0379195027 g=3 512 bits: p=85591083890203292422929753448312002013227700164244056883767436387780 3988137714894694780639823858404151944019992782250585325661689168497741 6276395927762259 g=3 1024 bits: p=10756096062425499020105845514001321706994933124811434157429824859705 5584898126321093330768462486995125563469663163251404817709163750190539 8111908385982976186475492023836046174671146008757104643109463540714363 4989690852152224155785251701387082100575086970999263664414942032660939 2283245879753842682719140993487 g=2 2048 bits: p=28401819833194672263220074318568239913240629340614134316461681527129 7400190276361407578313119949940155208450277590596255133024483132860231 2683364685437571458516690608818388566379408009734093619889676676688482 4330230759478149039933887325452487112982841213248410034360015394086822 8492443771741195501623918057531604439427869721645027989688343068984123 6647850119363273567137904693671110310979494733094653291727319950103948 4827099108375018229688415849095088940888827138097109161920916553046346 9800257941704054817481593649080741474714321422084101095199976587101477 59474207550469840261684005212634493995003979085986141372247 g=2Those numbers do not have to be calculated every time but in fact can be hard-coded in your application.
3D Vision for Embedded Systems
3D Vision is an integral part of every autonomous robot. Getting it right is not trivial and certainly not cheap. We struggled with this problem ourselves when trying to build an autonomous Mikrokopter drone. Cheap systems are not very reliable, good systems are disproportionately expensive and often very heavy. However I believe there may be hope yet for usable and cheap 3D vision systems for hobbyist and hackers alike. Granted, Microsoft is a rather unexpected reason for such hope but their Project Natal (now Kinect) might just be what we have been waiting for (image by litheon).
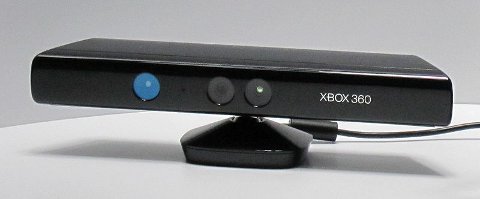
It’s a structured-light 3D scanner developed by PrimeSense. While being used for measuring the three-dimensional shape of an object I think it should be possible to use it as a 3D vision system for indoor robots. So I would really like to see hackers taking this cheap device apart and re-purposing it for home-brew 3D vision. In their paper “A low cost 3D scanner based on structured light“, Rocchini et al. describe how to build one and this incredible instructables article explains how to build one also and even provides source code.
Of course there are other exiting technologies out there, a comprehensive list of 3D vision devices can be found at acroname.com (including prices). The devices range from expensive but effective and impressive Time-of-flight cameras (vendors: [1] [2] [3]) to LIDAR systems. The latter were very successfully used in DARPA’s Urban Challenge autonomous driving competition. A very interesting video that describes how LIDAR works in detail is available at blip.tv.
Of course there’s also the good old ultrasonic and infrared sensors that are cheap and have low resolution and are moderately reliable but they can certainly be very useful.
Sphinx Cheatsheet
I find myself working with the Sphinx Documentation Generator a lot recently. It is a great tool to easily create intelligent and beautiful documentation and it is based on reStructuredText. I put together a cheat sheet of all the commands I frequently use. There are a number of very good ones out there ([1] [2] [3] [4]) but I find it more convenient to work with my own.
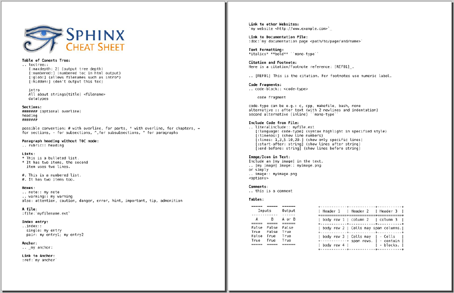
You can access the PDF from here and the source (OpenOffice Writer document) here. If you find it useful or would like an item added, feel free to leave a comment.
Infobox Icons
I like to scribble with Inkscape while listening to great music or radio plays to relax and clear my head. Today I created a couple of icons that I plan to use in documents to mark special boxes:
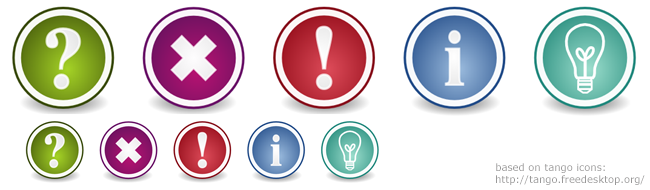
It’s a question, an error, a warning, an information and an idea icon. I currently use them in software documentation using the Shpinx Python Documentation Generator. It’s a very good tool to create beautiful documentation especially for software projects but I also use it to publish my game design document. Here’s an example of how the icons could be used:
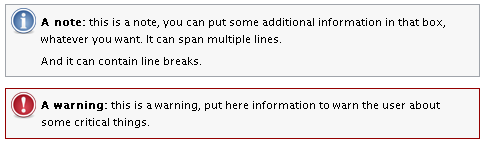
I based the icons upon images from the Tango Icon Gallery. You may download an archive containing the source (Inkscape .svg) and PNGs of different size here. Use them as you wish, if you find them useful I’d be very happy if you left a comment here.
Back to Browser Games
I’m back thinking about games, browser games to be exact. It’s been a while since I thought about games or wrote code for one – it’s been about 1 year since I stopped developing a prototype I called ‘Prototype 4’ which is a web based Master of Orion clone. I was working on the game full time for about a month but then had to stop because I had to take up a job to get some money and I also had to finish my diploma thesis. It progressed fairly far further than any other of my prototypes in fact: I created some nice graphics and a layout, a cool javascript user interface, a very efficient yet flexible back end library in PHP and a C++ battle calculator. Here are 2 screenshots of what the game looks like:
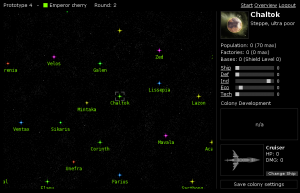
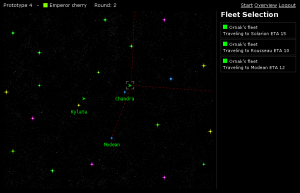
This was supposed to be a very literal copy of Master of Orion that you could play online with up to 15 other players on one map. Yet now I have something different in mind and I also have a different approach. It will only be a hobby project and I don’t plan to get immediate results, I want it to progress and evolve in my mind until I’m at a point where I can start writing code. Writing the code should be just handcraft at that point as I have most of the skills and knowledge to develop such a game.
What I’m doing now is writing a design document. The design will be highly influenced by the Foundation Series, one of the greatest science fiction series ever written. The first version of the design document can be found here. I still want the game to have a Master of Orion look and feel to it and it will certainly be a 4X type of game but I want different factions to be completely different (comparable to the different races in Starcraft) in the way they are played. I would like to see a “First Foundation” faction that relies on technology, economy and firepower and a “Second Foundation” faction that relies solely on the power of their minds, e.g. mind control. More about that can be found int the design document. A third faction is also possible but I have no concrete ideas about that yet. As the game design and the game itself progresses I will occasional write articles about it here on this blog.
Great Programming Quotes and Science Jokes
Today I stumbled across a great thread at Stack Overflow about great programming quotes. Here are the ones I liked most and therefore had to save:
On two occasions I have been asked, ‘Pray, Mr. Babbage, if you put into the machine wrong figures, will the right answers come out?’ I am not able rightly to apprehend the kind of confusion of ideas that could provoke such a question. Charles Babbage
Perfection is achieved, not when there is nothing more to add, but when there is nothing left to take away. Antoine de Saint Exupéry
To iterate is human, to recurse divine. L. Peter Deutsch
Don’t worry about people stealing your ideas. If it’s original, you’ll have to ram it down their throats. Howard Aiken
People who are really serious about software should make their own hardware. Alan Kay
And on a less serious note a colleague of mine told me a couple of hilarious science jokes from Brian Malow. Watch a video of the jokes here. My favourite:
A room temperature superconductor walks into a bar. The bartender says, “We don’t serve any superconductors in this bar.” The room temperature superconductor leaves without putting up any resistance.
Matlab C++ Integration
I’m currently working on integrating some Matlab Algorithms into a larger C++ project using the Matlab Compiler. It works rather well however I stumbled across a couple of quirks and caveats. First two facts that make integrating Matlab difficult: in Matlab indexes start at 1 and Matlab arrays are column-major ordered. As a result if you want to use data fields in both C/C++ and Matlab you have to transpose the fields. If the fields contain indexes you might also have to increment and decrement the values of the fields.
Another caveat I found is also related to array indexes. When using a Matlab function that takes as parameters matrix indexes you might think of creating an mwArray of type mxUINT32_CLASS. Wrong. You have to pass indices – as strange as it sounds – as doubles so you should use mxDOUBLE_CLASS.
One custom Matlab function I wanted to use in C++ took as an argument a cell array. A cell array is an array containing other arrays. In Matlab you create a cell array like this:
a = magic(5); b = ones(3); z{1} = a; z{2} = b;
If you look at z now you will get something like this:
z = [5x5 double] [3x3 double]
An array of arrays. Easy. Well if you want to construct a cell array in C++ you have to do something like this:
mwArray a(5, 5, mxDOUBLE_CLASS); mwArray b(3, 3, mxINT16_CLASS); mwArray z(1, 2, mxCELL_CLASS); z.Get(1,1).Set(a); // Sets z(1) = a z.Get(1,2).Set(b); // Sets z(2) = b
You can find the description on this page.
If you get used to those little quirks however the Matlab Compiler is a very powerful tool to include your crazy Matlab scripts in a stand alone C++ application. One of the next things I would like to investigate are free (open source) Matlab Compiler alternatives such as the Octave C++ integration and compiler.
Civilian autonomous and remote controlled drones
Here’s a quick summary of some of the most interesting autonomous and remote controlled drones out there today. The occasion is that I and 3 others have built a quadcopter drone last semester with the goal to fly it autonomously. While the time we had to finish the project was limited and our project did not reach full autonomous flight just yet we learned a lot about the system and the challenges of such an endeavor. One team member has made the project his diploma thesis and continues to work on the vehicle.
The two images show our drone dubbed B.I.R.D. (Basic intelligent research drone) in flight and the microcontrollers and sensors that control the drone. It is based on the the Mikrokopter project.
Another very interesting drone is the nano air vehicle by AeroVironment. It’s a flapping-wing vehicle for indoors use. Check out this amazing video to see it in action. I’m guessing that while the flapping-wing design is a lot more complicated than the brushless motors in our drone it is more energy efficient.
The third drone I would like to share was built by Armadillo Aerospace. They claim level 2 of the Northrop Grumman Lunar Lander Challenge with it. For more information read on here and be sure to check out this video. Notice how large this vehicle is (can be seen in the end of the video).
The fourth drone is really scary. And it’s not civilian at all. It’s called the Multiple Kill Vehicle and was supposed to shoot down intercontinental rockets in space. The project is apparently canceled but the video is impressive and scary nevertheless. Check it out here.
Update: Researchers at MIT’s Robust Robotics Group have developed a robotic helicopter capable of autonomously flying inside buildings or other GPS-denied environments. Here’s a very interesting video about their project. They achieved what we planned for our project – and I have to say it is very impressive and they did an excellent job.
Visualizing Multithreading
I’ve been pondering about the question of how to model and visualize multithreaded applications in a simple, efficient and aesthetic way. Naturally I’ve been looking into UML behavior diagrams first but I was not really satisfied with what I found. Now I am not an expert on UML but I know my way around class diagrams, activity diagrams, sequence diagram and state machines but they all did not seem to fit what I wanted to visualize.
I wanted to show multiple threads communicating and synchronizing with each other. I’ve read the argument that something like multithreading concerns only the implementation of a system and can thus be ignored when modeling. I agree with the argument however there will come a time in the development phase of a system when one needs to think about multithreading and one wants to sketch out how the system facilitates multiple threads to be efficient. And for that I have not found good tool within UML.
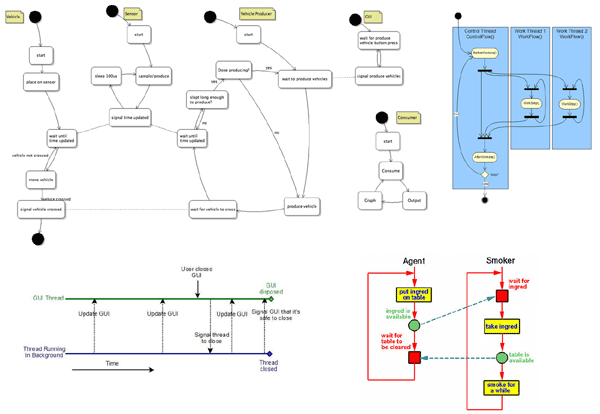
So I searched for examples of diagrams that visualize multithreading. The collage shows 4 different diagrams I found rather interesting. The first one is from this page about a traffic simulator written in Java (orignal image). It shows different threads and how they signal each other. It’s simple yet quite clear what is going an. The second image is taken from a CodeProject documentation about a genetic library (original image). It sort of uses UML symbols but it’s not clear what happens at the fork and join points. The way I figure it, those symbols inside the threads are supposed to be branches not forks. The third image is also from a CodeProject page – this time it’s a how-to about avoiding an exception when using multiple threads (original image). It reminds me of a sequence diagram. There are no blocking states in the diagram and I figure in this particular application the threads are non-blocking so diagram fits the application perfectly. The fourth diagram I liked the most. It’s from the Multithreaded Programming with ThreadMentor tutorial (original image) and has special symbols for sending signals and waiting for signals.
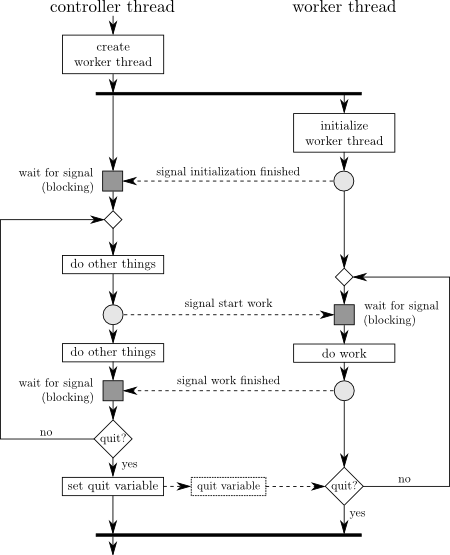
So I gave it a try myself and tried to model a simple controller and worker thread scenario based on the last of the example diagrams I found. The gray boxes and circle represent a signaling concept where one thread can send a signal and the other thread waits until it receives the signal. I also included a shared variable that handles the task shutdown. One thread can set the variable and the other branches depending on that variable. I am not happy yet with this particular part of the image. If anyone has a better idea or pointer to resources on that topic feel free to leave a comment.